Original Post: Tasting the mix of Python and SAP - Volume 3
Hello and welcome back to this little corner of Scripting Languages fun -:)
Today, we're going to see how can we use wxPython and SAP to make an SE16 emulation.
So, what's wxPython? It's a Python wrapper of the C++'s wxWidgets that allows us to create rich UI applications.
For this blog I was tempted to use Tkinter but gotta admit that I like wxPython more...as I have already used it in my Ruby projects. Anyway in Ruby it was a little bit easier than in Python -:P
Enough talk, let's go to the source code...(That could fit nice on a T-Shirt, right?)
Again, and as always in my Python/SAP projects...I used YAML to host the SAP connections parameters.
sap.yml
ashost: localhost
sysnr: "00"
client: "001"
lang: EN
trace: 1
loglevel: warn
SE16_wxPython.py
import wx
import sapnwrfc
import wx.grid as wxGrid
conn = ""
table = ""
class MyApp(wx.App):
def OnInit(self):
self.frame = MyFrame(None, title="Login")
self.SetTopWindow(self.frame)
self.frame.Show()
return True
class MyFrame(wx.Frame):
def __init__(self, parent, id=wx.ID_ANY, title="",
pos=wx.DefaultPosition, size=(210, 150),
style=wx.DEFAULT_FRAME_STYLE,
name="MyFrame"):
super(MyFrame, self).__init__(parent, id, title, pos,
size, style, name)
self.panel = wx.Panel(self)
self.text_font = wx.Font(12, wx.DEFAULT, wx.NORMAL, wx.NORMAL)
self.t_user = wx.StaticText(self.panel, -1, "User",
size=(40, 20), pos=(10, 12))
self.t_password = wx.StaticText(self.panel, -1, "Password",
size=(40, 20), pos=(10, 32))
self.t_user.SetFont(self.text_font)
self.t_password.SetFont(self.text_font)
self.user = wx.TextCtrl(self.panel, value="", pos=(90, 10))
self.password = wx.TextCtrl(self.panel, value="",
pos=(90, 30),
style=wx.TE_PASSWORD)
self.btnConnect = wx.Button(self.panel, label="Connect",
pos=(70, 80))
self.Bind(wx.EVT_BUTTON, self.OnButtonConnect,
self.btnConnect)
def OnButtonConnect(self, event):
global conn
user = self.user.GetValue()
password = self.password.GetValue()
sapnwrfc.base.config_location = "sap.yml"
sapnwrfc.base.load_config()
conn = sapnwrfc.base.rfc_connect({'user': user,
'passwd': password})
self.Close()
myGrid = GridFrame(None, title="SE16 Emulator")
myGrid.Show()
class GridFrame(wx.Frame):
def __init__(self, parent, id=wx.ID_ANY, title="",
pos=wx.DefaultPosition, size=(600, 400),
style=wx.DEFAULT_FRAME_STYLE,
name="GridFrame"):
super(GridFrame, self).__init__(parent, id, title, pos,
size, style, name)
self.panel = wx.Panel(self)
self.text_font = wx.Font(12, wx.DEFAULT, wx.NORMAL, wx.NORMAL)
self.t_table = wx.StaticText(self.panel, -1, "Table",
size=(40, 20), pos=(180, 12))
self.t_table.SetFont(self.text_font)
self.table = wx.TextCtrl(self.panel, value="", pos=(225, 10))
self.btnShow = wx.Button(self.panel, label="Show Table",
pos=(330, 10))
self.Bind(wx.EVT_BUTTON, self.OnButtonShow, self.btnShow)
def OnButtonShow(self, event):
global conn
table = self.table.GetValue()
fields = []
fields_name = []
func_disc = conn.discover("RFC_READ_TABLE")
func = func_disc.create_function_call()
func.QUERY_TABLE(str(table))
func.DELIMITER("|")
func.invoke()
data_fields = func.DATA.value
data_names = func.FIELDS.value
long_fields = len(func.DATA())
long_names = len(func.FIELDS())
for line in range(0, long_fields):
fields.append(data_fields[line]["WA"].strip())
for line in range(0, long_names):
fields_name.append(data_names[line]["FIELDNAME"].strip())
self.grid = wxGrid.Grid(self.panel, pos=(0, 40),
size=(853, 320))
self.grid.EnableEditing(False)
self.grid.CreateGrid(long_fields, long_names)
for line in range(0, long_names):
field_name = fields_name[line]
self.grid.SetColLabelValue(line, field_name)
for line_f in range(0, long_fields):
data_split = fields[line_f].split("|")
for line_n in range(0, long_names):
self.grid.SetCellValue(line_f, line_n,
data_split[line_n])
def onClose(self, event):
global conn
conn.close()
if __name__ == "__main__":
app = MyApp(False)
app.MainLoop()
Now, the images:
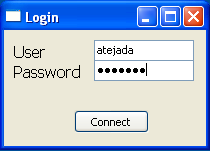
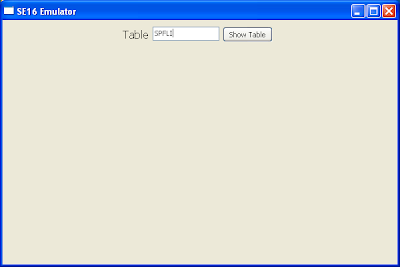
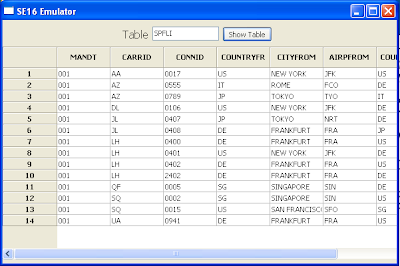
So basically, what we have here is a Login screen with the Username and Password and a connection button. When we connect to SAP, then the window dissapeared and a new window pops out. This new windows ask us for a table name and displays a grid containing all the information.
See ya next time -;)
Blag.
No hay comentarios:
Publicar un comentario